jQuery Selectors
Query Selectors are used to select and manipulate HTML elements. They are very important part of jQuery library. With jQuery selectors, you can find or select HTML elements based on their id, classes, attributes, types and much more from a DOM. In simple words, you can say that selectors are used to select one or more HTML elements using jQuery and once the element is selected then you can perform various operation on that. All jQuery selectors start with a dollor sign and parenthesis e.g. $(). It is known as the factory function.
Every jQuery selector start with thiis sign $(). This sign is known as the factory function. It uses the three basic building blocks while selecting an element in a given document.

Let's take a simple example to see the use of Tag selector. This would select all the elements with a tag name and the background color is set to "pink".
File: firstjquery.html
<!DOCTYPE html> <html> <head> <title>First jQuery Example</title> <script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/2.1.3/jquery.min.js"></script> <script type="text/javascript" language="javascript"> $(document).ready(function() { $("p").css("background-color", "pink"); }); </script> </head> <body> <p>This is the first paragraph.</p> <p>This is the second paragraph.</p> <p>This is the third paragraph.</p> </body> </html>
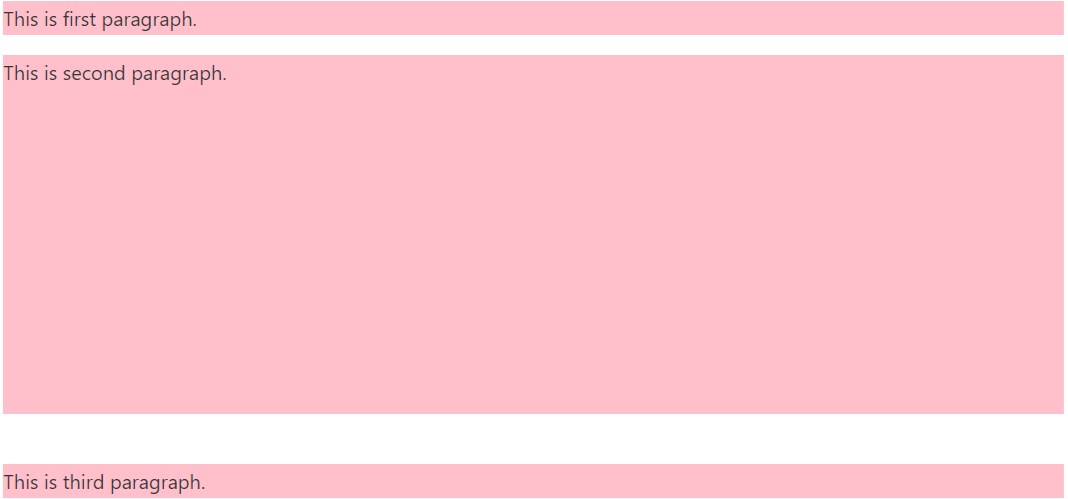