File Handling
Definition:
File handling in C++ is a mechanism to create and perform read/write operations on a file.File handling is used to store data permanently in a computer. Using file handling we can store our data in secondary memory (Hard disk).
We can access various file handling methods in C++ by importing the <fstream> class.
<fstream> includes two classes for file handling:
- ifstream:to read from a file.
- ofstream:to create/open and write to a file.
Example 1: Opening/Closing a file
#include <iostream> #include <fstream> using namespace std; int main() { // opening a text file for writing ofstream my_file("example.txt"); // close the file my_file.close(); return 0; }
This code will open and close the file example.txt.
Note: If there's no such file to open, ofstream my_file("example.txt"); will instead create a new file named example.txt.
Example 2: Read from a file
#include <iostream> #include <fstream> using namespace std; // open a text file for reading ifstream my_file("example.txt"); // check the file for errors if(!my_file) { cout << "Error: Unable to open the file." << endl; return 1; } // store the contents of the file in "line" string string line; // loop until the end of the text file while (!my_file.eof()) { // store the current line of the file // in the "line" variable getline(my_file, line); // print the line variable cout << line << endl; } // close the file my_file.close(); return 0; }
Suppose example.txt contains the following text:
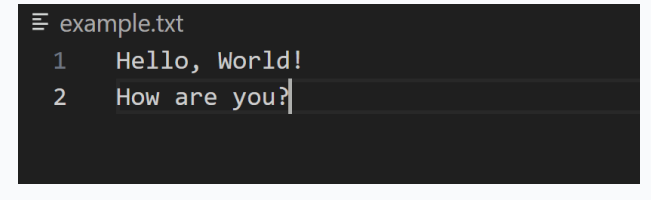
Then, our terminal will print the following output:
