C++ Function
Definition:
A function is a set of statements that takes input, does some specific computation, and produces output. The idea is to put some commonly or repeatedly done tasks together to make a function so that instead of writing the same code again and again for different inputs,we can call this function.
Types of Functions
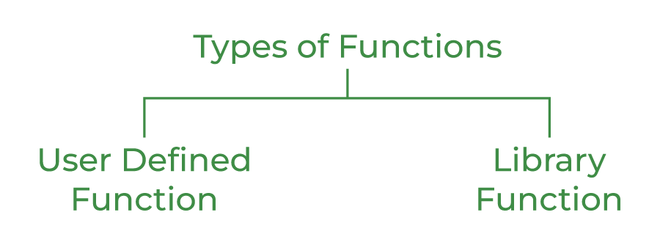
User Defined Function
User-defined functions are user/customer-defined blocks of code specially customized to reduce the complexity of big programs. They are also commonly known as “tailor-made functions” which are built only to satisfy the condition in which the user is facing issues meanwhile reducing the complexity of the whole program.
Library Function
Library functions are also called “built-in Functions“. These functions are part of a compiler package that is already defined and consists of a special function with special and different meanings. Built-in Function gives us an edge as we can directly use them without defining them whereas in the user-defined function we have to declare and define a function before using them.
Need of Functions
- Functions help us in reducing code redundancy. If functionality is performed at multiple places in software, then rather than writing the same code, again and again, we create a function and call it everywhere. This also helps in maintenance as we have to make changes in only one place if we make changes to the functionality in future.
- Functions make code modular. Consider a big file having many lines of code. It becomes really simple to read and use the code, if the code is divided into functions.
- Functions provide abstraction. For example, we can use library functions without worrying about their internal work.
Example 1:Function without parameters
#include <iostream> using namespace std; // declaring a function void greet() { cout << "Hello there!"; } int main() { // calling the function greet(); return 0; }
Output

Example 2:Function with parameters
// program to print a text #include <iostream> using namespace std; // display a number void displayNum(int n1, float n2) { cout << "The int number is " << n1; cout << "The double number is " << n2; } int main() { int num1 = 5; double num2 = 5.5; // calling the function displayNum(num1, num2); return 0; }
Output
